avl-rb-clojure-intro
Table of Contents
- 1 Intro to Clojure
- 1.1 What is Clojure?
- 1.2 Parts of Clojure
- 1.3 Immutability
- 1.4 Lazy Sequences
- 1.5 Function Definition
- 1.6 Function Definition
- 1.7 Reference Types
- 1.8 Let & Desctructuring
- 1.9 Let & Destructuring
- 1.10 REPL
- 1.11 Demo!
- 1.12 Pros/Cons
- 1.13 Who uses Clojure?
- 1.14 Resources
1 Intro to Clojure
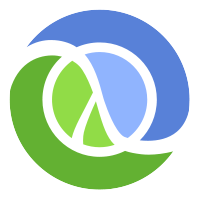
Toby Crawley
Asheville.rb
March 27, 2012
Asheville.rb
March 27, 2012
1.1 What is Clojure?
- A Lisp
- Homoiconic - Code as data
- Functional
- Not Object Oriented
- built around immutable data structures
- Runs on the JVM
- Embraces Java
- ClojureCLR, ClojureScript, clojure-py are alt. impls.
1.1.1 5 years old notes
- 1958
- s-expressions (symbolic)
1.2 Parts of Clojure
- very little syntax
1.2.1 Truthiness
nil
andfalse
are falsetrue
and everything else is true, including empty lists- same as ruby
1.2.2 Primitives - Strings
- Just java strings
"I'm a string"
1.2.2.1 No string interp notes
1.2.3 Primitives - Characters
- Just java characters
\a \b \x
1.2.3.1 but w/o single quotes notes
1.2.4 Primitives - Numbers
42 ;; long 42N ;; clojure.lang.BigInt 4.2 ;; double 4.2M ;; java.math.BigDecimal 22/7 ;; ratio
1.2.5 Primitives - Symbols
- names of things
str println clojure.string/split
1.2.6 Primitives - Keywords
- identifiers, offer fast equality
- often used as map keys
- are functions of maps
:foo :what-is-this-thing-in-my-hair? :some.namespace/bar (:key {:key "value"}) ;; => "value"
1.2.7 Collections - Lists
- implemented as a linked list
- have special eval semantics
(1 2 3 4) (str "a" "b" "c")
1.2.7.1 head is fast notes
1.2.8 Collections - Vectors
- an array
- generally used unless list semantics or performance needed
- functions of index
[1 2 3 4] ([1 2 3 4] 1) ;; => 2
1.2.8.1 tail is fast notes
1.2.9 Collections - Maps
- analogous to a Ruby Hash
- functions of keys
{:a :b, :c :d} ({:key "value"} :key) ;; => "value"
1.2.10 Collections - Sets
- functions of their members
#{1 2 3 4} (#{"yes" "no"} "yes") ;; => "yes" (#{"yes" "no"} "maybe") ;; => nil
1.3 Immutability
(def x {:a :b}) (assoc x :c :d) ;; => {:a :b, :c :d} x ;; => {:a :b} (def y [1 2 3]) (conj y 4) ;; => [1 2 3 4] y ;; => [1 2 3]
1.3.1 def creates a 'var' notes
- internal structure is shared
- makes concurrency easier
1.4 Lazy Sequences
- values in the sequence aren't realized until needed
- allows working with large/infinite datasets
(take 10 (range)) ;; => (0 1 2 3 4 5 6 7 8 9)
1.4.1 range is infinite notes
- take itself returns a lazy sequence
1.5 Function Definition
- named
(defn ham-is-good [a b] (println (if (or (= a "ham") (= b "ham")) "Ham goes good with anything!" (str a " and " b "? Grody!"))))
1.5.1 this is just data notes
1.6 Function Definition
- anonymous - lambdas
(fn [v] (println "I got" v)) #(println "I got" %)
1.6.1 compare to ruby blocks notes
1.7 Reference Types
- allow for controlled mutability
- can only be manipulated via special functions
- mutation occurs within a transaction
- must be explicitly dereferenced
(def an-atom (atom 0)) (swap! an-atom inc) @an-atom ;; => 1
1.7.1 STM & MVCC notes
- atoms - single changing value
- agents - asynchronous update of a single value
- refs - mutliple coordinated changing values; explicit (dosync)
1.8 Let & Desctructuring
- bind values in a closure
(let [full-name (some-name-producing-function) [first-name last-name] (split full-name #" ")] (println full-name "splits into" first-name last-name))
1.8.1 values only bound inside let notes
- introduces destructuring
- aka structural binding
1.9 Let & Destructuring
(let [point [123 456] [x-coord y-coord] point] (defn draw-something [height width {:keys [color opacity] :as options}] (draw-square x-coord y-coord height width color opacity) (something-else-that-wants-options options)))
1.10 REPL
- the Read-Eval-Print Loop
1.10.1 think irb notes
1.11 Demo!
1.12 Pros/Cons
- pros:
- write code with less errors
- bend your brain
- access to all Java libraries
- safe(r) concurrency
- cons:
- have to learn Java as well
- young ecosystem
1.13 Who uses Clojure?
- Heroku
- Datomic
- World Singles
- Sonian
- Runa
- Me! (therefore Red Hat)
1.14 Resources
- Clojure Koans - https://github.com/functional-koans/clojure-koans
- 4clojure - http://www.4clojure.com/
- #clojure on freenode
- clojure@googlegroups.com
- books
- Programming Clojure - Stuart Halloway
- Joy of Clojure - Michael Fogus & Chris Houser